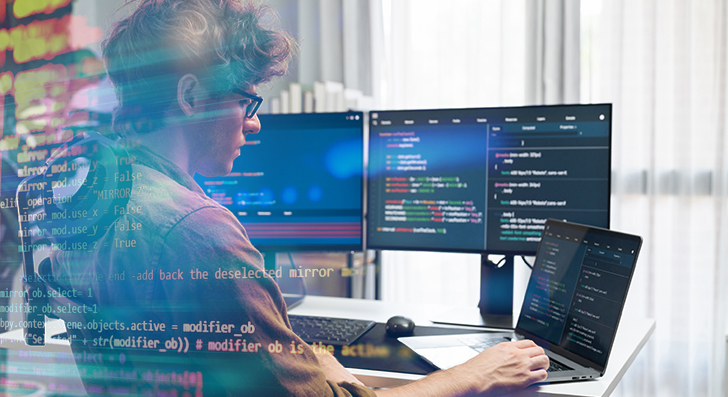
Scalability implies your software can cope with progress—more people, far more info, and even more visitors—without breaking. To be a developer, constructing with scalability in mind saves time and strain later on. In this article’s a transparent and sensible tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your prepare from the start. Several purposes are unsuccessful after they mature quickly because the initial structure can’t manage the additional load. As being a developer, you need to Consider early regarding how your method will behave stressed.
Get started by developing your architecture being flexible. Keep away from monolithic codebases where by anything is tightly connected. As an alternative, use modular structure or microservices. These patterns break your application into lesser, independent elements. Just about every module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your databases from day a single. Will it need to have to handle 1,000,000 end users or merely 100? Pick the correct sort—relational or NoSQL—determined by how your facts will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them however.
One more significant issue is to avoid hardcoding assumptions. Don’t write code that only functions underneath present-day disorders. Think about what would occur In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design patterns that assistance scaling, like message queues or occasion-driven systems. These assist your app handle much more requests with out receiving overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A perfectly-prepared technique is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the proper Databases
Deciding on the ideal databases can be a crucial part of setting up scalable apps. Not all databases are developed the same, and utilizing the Mistaken one can slow you down or maybe lead to failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If Of course, a relational database like PostgreSQL or MySQL is a great suit. They are solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to take care of far more traffic and knowledge.
In case your facts is more versatile—like person activity logs, products catalogs, or documents—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and generate designs. Are you carrying out numerous reads with fewer writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that may take care of higher compose throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You may not want Innovative scaling capabilities now, but deciding on a databases that supports them means you won’t require to change later on.
Use indexing to speed up queries. Keep away from unnecessary joins. Normalize or denormalize your information based on your accessibility patterns. And often check database functionality while you increase.
Briefly, the best databases depends on your application’s composition, velocity desires, And exactly how you hope it to mature. Choose time to select correctly—it’ll help save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each individual smaller hold off adds up. Poorly written code or unoptimized queries can decelerate performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Next, check out your database queries. These generally slow things down in excess of the code itself. Ensure that Each and every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and instead decide on certain fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
Should you see exactly the same knowledge being requested over and over, use caching. Shop the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with 1 million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to manage additional people plus more targeted visitors. If everything goes through one server, it will immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to one particular server carrying out all of the function, the load balancer routes users to distinctive servers based upon availability. This suggests no solitary server will get overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused promptly. When end users request exactly the same information and facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two prevalent varieties of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers databases load, improves velocity, and would make your app far more efficient.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you would like resources that allow your app grow effortlessly. That’s the place cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t must get components or guess future capacity. When visitors raises, you'll be able to incorporate a lot more assets with only a few clicks or routinely employing car-scaling. When targeted traffic drops, it is more info possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You could deal with making your application as an alternative to controlling infrastructure.
Containers are An additional important tool. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also help it become very easy to separate aspects of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, employing cloud and container resources suggests you are able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you would like your application to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when items go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better decisions as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application too. Keep an eye on how long it takes for customers to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, When your response time goes previously mentioned a limit or perhaps a services goes down, you should get notified immediately. This allows you take care of challenges rapid, typically just before consumers even discover.
Checking is likewise valuable if you make modifications. In the event you deploy a new element and find out a spike in mistakes or slowdowns, you can roll it again in advance of it brings about actual damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the right instruments in place, you keep in control.
Briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your procedure and making certain it really works effectively, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to build apps that improve smoothly with no breaking stressed. Begin modest, Imagine large, and Make wise.